Formatting R Code
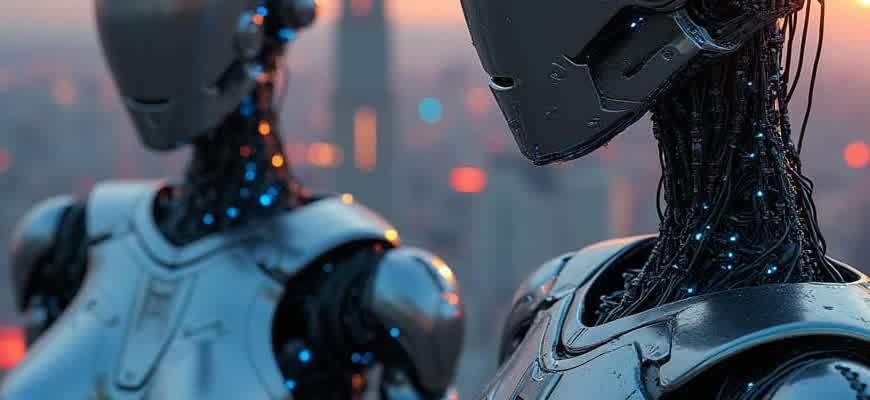
When working with R programming for cryptocurrency analysis, it's essential to maintain a clear and efficient code structure. Well-organized code not only improves readability but also ensures that scripts can be easily updated and debugged. This is especially important when dealing with large datasets from blockchain networks or financial exchanges.
Key Principles:
- Consistency in naming conventions
- Proper indentation to enhance readability
- Commenting and documentation for complex algorithms
To streamline your R code, consider using these practices:
- Use meaningful variable names to avoid confusion during analysis.
- Follow a consistent style for functions and loops.
- Break down long lines of code into smaller, manageable chunks.
Important: Consistent formatting helps avoid errors when working with external APIs, like those used for real-time cryptocurrency data feeds.
Here's a simple example of formatting a script that pulls cryptocurrency data from an API:
Step | Description |
---|---|
1 | Load necessary libraries (e.g., 'httr' for HTTP requests) |
2 | Set up API request parameters and handle responses |
3 | Format data for analysis (e.g., convert JSON to data frame) |
How to Enhance the Formatting of Your R Code for Better Readability
Formatting your R code is crucial for improving its readability and maintainability, especially when working with complex data analyses or when collaborating with others in the cryptocurrency domain. Clear and consistent formatting helps prevent errors and makes the codebase easier to navigate. By following a few best practices, you can enhance the overall structure of your code, making it more efficient and comprehensible.
One of the first steps in improving your code formatting is adhering to a consistent style. In the world of cryptocurrency analysis, where data manipulation and modeling can get complex, it's important to keep your R scripts neat and readable. This can be achieved by aligning function arguments, using appropriate indentation, and structuring your code into logical blocks.
Key Formatting Tips for Better R Code
- Indentation: Use consistent indentation (2 or 4 spaces) for nested code blocks. This helps distinguish different levels of logic within your script.
- Function and Variable Naming: Use meaningful and descriptive names for functions and variables. For example, instead of generic names like "data1", use something like "crypto_prices".
- Spacing: Add spaces around operators (e.g., +, -, *, /) to make expressions easier to read.
Example of Well-Formatted Code
# Loading necessary libraries library(quantmod) library(tidyr) # Fetching Bitcoin data btc_data <- getSymbols("BTC-USD", src = "yahoo", auto.assign = FALSE) btc_prices <- Cl(btc_data) btc_prices_clean <- na.omit(btc_prices) # Plotting Bitcoin price over time plot(btc_prices_clean, main = "Bitcoin Price Over Time", col = "blue")
Important Considerations
Always document your code clearly. In the context of cryptocurrency, it's essential to note the sources of your data and any transformations you apply to it.
Common Formatting Mistakes
- Inconsistent Spacing: Failing to use spaces around operators or after commas can make code harder to parse.
- Lack of Comments: Skipping comments in complex sections, such as data transformations, can make it difficult for others to understand your logic.
- Overcomplicating Code: Avoid writing overly complex one-liners. Break down complex logic into smaller, more understandable pieces.
Summary of Good Practices
Good Practice | Benefit |
---|---|
Consistent indentation | Improves readability and structure |
Descriptive variable names | Enhances code clarity |
Regular commenting | Helps others understand your approach |
Why Consistent Code Structure is Crucial for R Projects
In the world of cryptocurrency analysis, R is often the go-to tool for processing and visualizing vast amounts of financial data. When working with cryptocurrency data, it is essential to maintain a clean and well-structured codebase. Proper formatting can significantly enhance both the readability and functionality of your scripts, especially when dealing with complex market trends and large datasets. This is particularly relevant when working collaboratively in the field, where clear, consistent code can prevent errors and speed up debugging.
Moreover, given the dynamic nature of the cryptocurrency market, R scripts often require rapid adjustments. A well-organized and neatly formatted script reduces the learning curve when revisiting or updating the code in the future. By adhering to formatting standards, you not only ensure your code is more understandable but also facilitate smooth collaboration with others in the crypto space, making project scalability and team involvement easier.
Key Reasons for Maintaining Consistency in Code Formatting
- Improved Collaboration: A uniform code structure makes it easier for teams to collaborate, especially when working with large datasets like Bitcoin price trends or Ethereum gas fee data.
- Faster Debugging: Code that follows a standardized format is simpler to review, track issues, and implement fixes, saving time during critical moments, such as price volatility analysis.
- Enhanced Readability: Cryptocurrency analytics often involve complex mathematical functions. A well-formatted script allows even those unfamiliar with specific code blocks to follow the logic more easily.
Best Practices in Code Formatting for R Projects
- Consistently use indentation to signify code blocks, making the flow of logic clear.
- Apply descriptive variable names such as btc_price or eth_volume instead of generic names like x or y.
- Use comments liberally to explain important sections of code, especially when analyzing complex financial indicators or integrating with APIs for real-time data.
Effective formatting reduces the risk of misunderstandings, allowing team members to quickly spot the purpose of each function or section in your cryptocurrency analysis scripts.
Example of Well-Formatted Code
Step | Action |
---|---|
1 | Load necessary libraries like quantmod for financial data. |
2 | Import and clean cryptocurrency data using clear and descriptive function names. |
3 | Perform analysis on market trends with well-commented code for future reference. |
Step-by-Step Guide for Using `styler` with R Code
When working with R, maintaining a clean and readable code is essential, especially when dealing with complex data analysis or cryptocurrency projects. One effective tool to ensure code consistency and readability is the `styler` package. By applying automatic formatting to your code, `styler` helps make your code more structured, improving collaboration among data scientists and developers in the cryptocurrency domain.
In this guide, we’ll walk you through how to use `styler` to format your R code, making it easier to follow and align with best practices. Whether you are analyzing Bitcoin price trends or building a cryptocurrency prediction model, well-formatted code ensures clarity and reduces the likelihood of errors.
Getting Started with `styler`
To begin, you need to install the `styler` package if you haven’t already. You can do this by running the following command in your R console:
install.packages("styler")
Once installed, you can load it with:
library(styler)
Formatting Your Code
The primary function of `styler` is to automatically format your code according to predefined rules. To apply it to your R script, use the following command:
styler::style_file("your_script.R")
This command will style the entire script, improving indentation, spacing, and aligning code structures. Let’s break down a few examples to see how the formatting helps in the cryptocurrency analysis context:
- Aligning parameters in functions, which is useful when setting up variables like Bitcoin prices or transaction volumes.
- Ensuring consistent use of spaces, making your analysis of Ethereum data sets easier to read.
- Standardizing the use of operators, such as `<-` for assignment, which reduces confusion during collaborative cryptocurrency projects.
Advanced Usage of `styler`
For more control over the formatting, you can specify particular styling rules by using the style_text function:
styler::style_text("x <- c(1, 2, 3, 4, 5)")
This allows you to apply styling to specific parts of your code, such as functions related to blockchain transactions. You can also use the `style_file` command in combination with version control tools to keep your codebase tidy across multiple contributors.
Formatting Cryptocurrency Code Example
Unformatted Code | Formatted Code |
---|---|
btc_data<-read.csv('btc_prices.csv') |
btc_data <- read.csv('btc_prices.csv') |
predict_btc<-function(x){ return(x*1000) } |
predict_btc <- function(x) { return(x * 1000) } |
Important Notes
Remember that while `styler` greatly improves code readability, it should be used in conjunction with proper version control systems (e.g., Git) to ensure that formatting changes do not interfere with your collaborative workflow in cryptocurrency-related projects.
By adopting `styler`, you not only improve the visual aspect of your R code but also enhance the overall development process, especially when analyzing cryptocurrency data, ensuring that your models and analyses are both accurate and understandable.
Integrating R Code Formatting in Cryptocurrency Version Control Workflows
In the fast-evolving world of cryptocurrency analysis and development, maintaining clean and readable R code is crucial for collaborative work. When working with version control systems (VCS) like Git, the integration of automated formatting tools can significantly improve code quality and team productivity. This practice ensures that developers focus on functionality, while the formatting is consistent across all project files, reducing time spent on trivial code review comments.
Automated R code formatting tools, such as *styler* and *formatR*, can be seamlessly integrated into your version control process. These tools enforce consistent styling across your R scripts, ensuring that every commit adheres to predefined formatting rules. This is especially important in cryptocurrency projects where analyzing large datasets and performing complex calculations can lead to messy, hard-to-read code if not properly maintained.
Best Practices for Code Formatting in Version Control
- Ensure all contributors use the same formatting tool to maintain consistency in the codebase.
- Integrate formatting checks into the CI/CD pipeline to automatically enforce code style rules on each commit.
- Make use of pre-commit hooks to format the code before every commit, reducing errors and manual effort.
For teams working on cryptocurrency analytics, implementing code formatting can avoid issues such as inconsistent indentation, improper spacing, and unreadable function definitions. Below is an example of how this can be achieved through Git hooks:
Tip: Use the *pre-commit* framework to set up automatic R code formatting when changes are committed to your repository. This ensures every commit includes correctly formatted code.
Sample Workflow for Cryptocurrency Projects
- Install *styler* or *formatR* in your R environment.
- Set up pre-commit hooks to format R code automatically on every commit.
- Integrate CI/CD tools like GitHub Actions or GitLab CI to run the formatting checks during pull requests.
This workflow ensures that your R code is not only readable but also ready for deployment, preventing formatting issues from causing delays in cryptocurrency algorithmic trading systems or blockchain analysis tools.
Tool | Integration Type | Benefits |
---|---|---|
styler | Automated formatting | Consistent code style across the team |
pre-commit | Pre-commit hook | Prevents formatting issues before commits |
CI/CD | Continuous integration | Ensures all pull requests comply with formatting standards |
Best Practices for Consistent R Code Formatting Across Teams
When developing cryptocurrency analysis tools in R, maintaining a consistent code style is essential for collaboration, especially across different teams. Whether you are working on a decentralized finance (DeFi) algorithm or a trading bot, clear and uniform code makes it easier to track changes, understand logic, and debug issues. Adopting a set of formatting rules ensures that developers can quickly integrate code changes without misunderstanding variable usage or function definitions.
By adhering to a unified formatting strategy, team members can focus on solving complex problems, like predicting market trends or optimizing portfolio management, rather than wasting time on deciphering each other's code. Let's explore some of the best practices that can help keep R code consistently formatted in cryptocurrency-related projects.
Key Formatting Guidelines
- Indentation: Always use 2 spaces for indentation. This keeps the code visually compact and avoids the issues that arise from mixing spaces and tabs.
- Line Length: Limit lines to 80 characters to prevent horizontal scrolling when working on long formulas or queries related to market data.
- Function Naming: Use clear, descriptive names with lowerCamelCase. This ensures functions like "calculateRisk" or "fetchMarketData" are easy to identify and understand.
- Comments: Provide concise comments explaining complex parts of code, especially in areas dealing with crypto data preprocessing or high-frequency trading strategies.
Tools to Help Standardize Formatting
- lintr: A static code analysis tool that checks for formatting issues and coding style violations. It can be integrated into CI pipelines to ensure consistent style in pull requests.
- styler: A package that automatically formats R code according to pre-defined style guides, helping teams enforce consistency without manual effort.
- RStudio Addins: Customize RStudio with formatting tools that can be triggered with a single click to standardize code style across all team members.
Consistency in R code formatting can significantly enhance team collaboration and productivity. This practice is essential when working with data-rich, rapidly evolving fields like cryptocurrency trading algorithms.
Formatting Standards Table
Practice | Recommendation |
---|---|
Indentation | 2 spaces |
Line Length | 80 characters |
Function Naming | LowerCamelCase |
Commenting | Brief, meaningful comments for complex code |