Professional C Programming
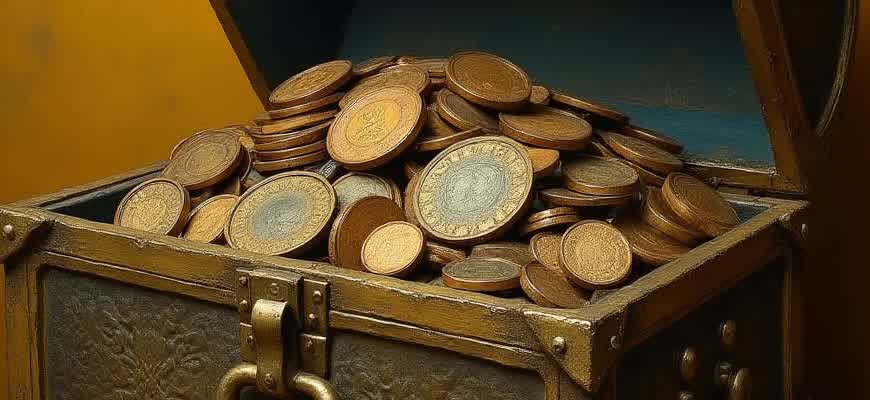
When diving into the world of cryptocurrencies, a deep understanding of low-level programming is essential. One of the most powerful languages for building blockchain applications is C, due to its efficiency and performance. In this context, developers must hone their C programming skills to ensure optimal execution of complex algorithms, network protocols, and security systems that power decentralized platforms.
Here are some key areas where C programming plays a crucial role in cryptocurrency development:
- Memory Management: C allows direct manipulation of memory, which is critical for performance in decentralized applications.
- Data Structures: Efficient management of linked lists, trees, and hash tables is necessary for storing blockchain data.
- Low-Level Networking: C provides the tools to build fast and secure network layers for peer-to-peer transactions.
Key technical skills include:
- Understanding pointers and memory allocation.
- Developing efficient hashing algorithms.
- Implementing cryptographic functions securely.
Note: Mastery of C programming is not just about syntax. It's about understanding the underlying concepts that drive blockchain technology, such as distributed ledgers and consensus mechanisms.
The table below highlights some of the important features of C that are vital for cryptocurrency projects:
Feature | Importance in Cryptocurrency |
---|---|
Pointer Arithmetic | Crucial for efficient memory management in blockchain nodes. |
Low-level System Access | Allows interaction with hardware for optimized performance. |
Bitwise Operations | Used in hashing, cryptography, and transaction validation. |
Mastering Memory Allocation in C for Cryptocurrency Applications
In the world of cryptocurrency, applications must handle massive volumes of data efficiently, making memory management in C crucial for building secure, high-performance systems. When working with cryptocurrencies, such as blockchain protocols, wallet software, or transaction processing systems, understanding low-level memory manipulation can prevent performance bottlenecks and security vulnerabilities. Robust memory handling ensures that applications can scale, reduce resource consumption, and handle unpredictable loads while maintaining reliability.
Memory management in C involves allocation, deallocation, and proper error handling, all of which are critical in developing secure and efficient systems. For cryptocurrency software, improper memory handling can lead to crashes, data corruption, or even security exploits. By mastering these principles, developers can create applications that are not only fast but also safe from common pitfalls such as buffer overflows and memory leaks.
Key Techniques in Memory Management
- Dynamic Allocation: Functions like malloc, calloc, and realloc allow developers to allocate memory dynamically at runtime, which is essential for handling the unpredictable data sizes in cryptocurrency applications.
- Memory Deallocation: Properly freeing allocated memory using free ensures that the system does not waste resources or suffer from memory leaks, which is particularly important in applications with long runtimes and heavy data processing.
- Error Checking: Always check the return value of memory allocation functions to avoid using null pointers, which can lead to crashes or undefined behavior.
Best Practices
- Use Smart Pointers and Memory Pools: For high-performance applications, especially those dealing with numerous transactions, memory pools and smart pointers (though C lacks them natively) can offer optimized solutions to avoid frequent allocation/deallocation.
- Segregate Memory for Sensitive Data: Cryptocurrency applications often deal with sensitive information like private keys. Isolate memory areas that store sensitive data and apply encryption before deallocation.
- Ensure Memory Bounds Safety: Always be mindful of buffer sizes, especially in cryptographic operations where large data chunks are processed. Ensure that buffers are properly sized to avoid overflow vulnerabilities.
Memory Allocation Breakdown for Cryptocurrency Systems
Operation | Function | Use Case |
---|---|---|
Allocate Memory | malloc(), calloc() | Used to allocate memory dynamically for storing large blocks of transaction data or blockchain blocks. |
Reallocate Memory | realloc() | Adjust memory allocation dynamically as more transaction data is received, useful in handling varying amounts of blockchain data. |
Free Memory | free() | Deallocates memory after use, critical for avoiding memory leaks in long-running cryptocurrency services. |
Proper memory management is not just about allocation and deallocation. In the context of cryptocurrency systems, it is about ensuring data integrity, avoiding security risks, and maintaining the efficiency needed to handle millions of transactions per second.
Optimizing C Code for Speed and Low Latency in Cryptocurrency Applications
When developing cryptocurrency applications, optimizing C code for speed and low latency is crucial to handle the demands of real-time transactions and high-frequency trading. Optimizing code can significantly improve performance, reduce processing times, and minimize delays in transaction processing. This is especially important in a highly competitive environment like blockchain and cryptocurrency, where milliseconds matter.
One effective strategy for optimization is reducing unnecessary memory allocations and avoiding costly dynamic memory operations. By using efficient algorithms and focusing on the system's cache architecture, C developers can minimize the number of CPU cycles required for each operation. Below are some critical techniques to keep in mind for improving the speed and responsiveness of your C code.
Key Optimization Techniques
- Efficient Memory Management: Always allocate memory in blocks and avoid unnecessary allocations in critical sections of your code.
- Algorithm Selection: Choose algorithms with better time complexity to reduce processing time. For example, use hash-based methods for fast lookups in blockchain validation.
- Minimize Context Switching: Reduce the number of threads or processes involved in time-sensitive operations to avoid costly context switches.
- Use Fixed-Point Arithmetic: In scenarios involving cryptocurrency transactions, floating-point operations can be slow. Fixed-point arithmetic can offer faster, more predictable performance.
Note: Minimizing latency in real-time transaction processing is key to ensuring a seamless user experience in cryptocurrency platforms.
Performance Metrics and Tools
To track the performance improvements after optimization, you can use the following tools:
- gprof: A performance analysis tool that helps identify slow functions in your code.
- Valgrind: A tool for memory profiling and detecting memory leaks, crucial for optimizing memory usage.
- Perf: A performance monitoring tool that helps track low-level hardware events and software performance issues.
Important Performance Factors
Factor | Impact |
---|---|
Cache Locality | Improves memory access speed and reduces latency by keeping frequently accessed data close to the processor. |
Parallel Processing | Using multiple threads or cores for computation can drastically reduce latency and improve throughput in multi-user environments. |
Loop Unrolling | Reducing the number of iterations in critical loops can lead to performance improvements by minimizing control overhead. |
Effective Debugging Techniques for C Code in Cryptocurrency Development
In the realm of cryptocurrency development, maintaining the integrity of C code is crucial to ensure secure transactions, minimize errors, and optimize performance. Debugging is a vital skill that every developer must master to address bugs promptly and efficiently, especially in systems where precision is paramount. Cryptographic algorithms, blockchain technologies, and decentralized applications often rely on C for their low-level operations, making it essential to address any issues that arise swiftly to prevent larger security vulnerabilities or performance degradation.
When debugging C code within cryptocurrency systems, developers need strategies that not only fix the immediate problem but also ensure long-term stability and scalability of the system. This requires a deep understanding of both the logic and the underlying infrastructure of the software. By applying systematic debugging methods, developers can efficiently pinpoint bugs and resolve them with minimal downtime.
Key Debugging Strategies for Cryptocurrency C Code
- Use of Debugging Tools: Tools like GDB (GNU Debugger) and Valgrind can help identify memory leaks, buffer overflows, and segmentation faults. These tools allow developers to step through code, observe variable states, and track down issues at runtime.
- Test Cases and Unit Testing: Implementing automated tests ensures that the codebase remains reliable. In cryptocurrency applications, unit tests can verify cryptographic functions and validate blockchain logic, making it easier to catch potential issues early.
- Log Analysis: Comprehensive logging mechanisms are essential in identifying where the code breaks down. Detailed logs help developers track unexpected behavior and fix issues based on real-time data.
Steps for Efficient Bug Fixing
- Reproduce the Issue: Before fixing a bug, it's essential to reproduce the issue consistently. Understanding the exact scenario in which the bug occurs helps target the root cause.
- Analyze Stack Traces: Stack traces can provide a wealth of information about the exact point of failure. By examining the trace, developers can quickly isolate problematic functions or variables.
- Fix and Test: After identifying the bug, implement a solution and run thorough tests to ensure the fix works across all use cases, especially in high-load or edge scenarios often encountered in blockchain operations.
Common Issues in C Cryptocurrency Code and Their Solutions
Issue | Solution |
---|---|
Memory Leaks | Use tools like Valgrind to detect and fix memory leaks by ensuring all allocated memory is properly freed. |
Buffer Overflows | Implement bounds checking to avoid overflows, especially in cryptographic algorithms where precise data handling is crucial. |
Segmentation Faults | Utilize GDB to track down the source of segmentation faults, which often arise due to invalid memory access or pointer dereferencing. |
Important: In cryptocurrency codebases, errors such as memory corruption or invalid transaction handling can lead to severe security risks. Fast and accurate debugging techniques not only save time but also enhance the system’s robustness.
Deep Dive into Pointers and Dynamic Memory Management with Cryptocurrency Context
In the world of cryptocurrencies, efficient memory management can directly impact performance, especially in scenarios where quick access to large datasets is crucial, such as blockchain validation or transaction processing. Understanding pointers and dynamic memory allocation is a fundamental concept in C programming that plays a key role in optimizing these processes. Just as a blockchain requires careful tracking of blocks in memory, pointers allow developers to dynamically manage the data they need at runtime, rather than relying on static, predefined structures.
Pointers are variables that store memory addresses, which means that instead of directly storing data like an integer or a string, they store the address of where the data is located in memory. This concept is particularly relevant in cryptocurrency applications where resources, such as network data or transaction information, are continuously changing and need to be managed efficiently without overburdening system memory.
Understanding Dynamic Memory Allocation
Dynamic memory allocation allows programs to allocate memory at runtime using functions like malloc(), calloc(), and free(). This flexibility is critical when dealing with large, unpredictable datasets, like a cryptocurrency wallet or a node's transaction history. For example, instead of allocating a fixed-size array for storing transactions, dynamic memory allocation allows a system to expand or shrink memory usage based on the real-time needs of the program.
In the context of cryptocurrencies, dynamic memory management ensures that applications can handle increasing user activity or fluctuating blockchain sizes without crashing or consuming unnecessary system resources.
- malloc() allocates a specified number of bytes of memory.
- calloc() allocates memory and initializes it to zero.
- free() deallocates previously allocated memory to avoid memory leaks.
Let’s consider a simple example where memory needs to be allocated for storing a variable-length list of blockchain transactions:
Function | Action |
---|---|
malloc() | Allocates a block of memory for storing a set number of transactions. |
free() | Releases memory that was used for the transactions once they are no longer needed. |
In this example, the ability to allocate and release memory dynamically ensures that cryptocurrency software remains flexible and responsive under varying loads, preventing unnecessary memory consumption and improving overall performance.
Advanced C Programming: Data Structures and Algorithms in Cryptocurrency
In the context of cryptocurrency systems, understanding the efficient use of data structures and algorithms is critical. Given the need for high-performance transaction processing and robust blockchain networks, mastering these techniques in C can lead to significant improvements in the scalability and security of crypto-based applications.
The key to optimizing performance lies in selecting the right data structures. For example, using hash tables and linked lists can provide faster access to transactions or blockchain data, minimizing latency during real-time cryptocurrency exchanges. With C, developers can implement custom data structures that cater to specific needs, reducing overhead and maximizing throughput.
Common Data Structures in Cryptocurrency Development
- Hash Tables: Crucial for fast lookups, enabling quick validation of transactions and blocks in a blockchain.
- Merkle Trees: Efficiently summarize large sets of data (e.g., transactions) to ensure data integrity and improve security.
- Linked Lists: Used in blockchains to link blocks in a sequential manner for easier traversal and verification.
Optimizing these structures for performance can be achieved by employing advanced algorithms. For example, utilizing sorting algorithms for transaction queues or efficient searching methods such as binary search trees can greatly speed up the consensus process and reduce network load in a cryptocurrency system.
Effective algorithmic design is key to ensuring that a cryptocurrency application remains responsive, even during periods of high network activity.
Example: Blockchain Data Structure
Block Number | Hash | Previous Hash | Data |
---|---|---|---|
1 | abc123 | 0 | First Block |
2 | def456 | abc123 | Transaction Data |
In this table, each block in the blockchain is linked by its hash and the hash of the previous block, forming a chain that can be easily traversed or verified. Using efficient traversal algorithms for these data structures ensures that the blockchain remains consistent and secure across all nodes in the network.
Integrating C with Other Languages: Use Cases in Cryptocurrency Development
Integrating C with other programming languages can significantly enhance the performance and functionality of cryptocurrency-related applications. C is known for its speed and efficiency, making it a popular choice for developing critical components such as blockchain algorithms, encryption mechanisms, and networking protocols. When combined with other languages like Python, JavaScript, or Rust, developers can leverage the best features of each language for specific tasks, optimizing the overall system. This integration is especially useful in high-performance environments where low-level system operations and high-level scripting need to work together seamlessly.
Practical examples of using C in cryptocurrency projects include the development of mining algorithms, cryptographic libraries, and transaction processing systems. By combining C with languages such as Python, the complex logic and heavy computations can be offloaded to C, while Python can handle higher-level tasks like scripting and interacting with APIs. This hybrid approach allows developers to take advantage of the unique strengths of both languages, ensuring both efficiency and flexibility.
Common Use Cases
- Mining Algorithms: C is used for implementing the core mining logic, while Python or JavaScript can manage user interfaces or handle communication between miners.
- Cryptographic Libraries: C provides high-speed cryptographic operations, while Rust or Go can be used to build secure and concurrent APIs for interacting with these libraries.
- Transaction Validation: C ensures quick transaction verification in blockchain networks, with higher-level languages like Python handling transaction management and data visualization.
Example Table: C and Python Integration
Language | Use Case |
---|---|
C | Cryptographic operations (hashing, encryption) |
Python | Transaction management, Blockchain interaction |
Integrating C with other languages helps in offloading performance-critical tasks, providing a balance between speed and flexibility in cryptocurrency projects.
Building Cross-Platform C Applications: Tools and Best Practices
When developing cross-platform C applications, it's essential to ensure compatibility across various operating systems without compromising performance. This becomes even more crucial in the context of cryptocurrencies, where security and efficiency are key. To achieve this, using the right tools and following best practices is critical for building applications that run seamlessly on multiple platforms like Windows, macOS, and Linux.
One of the most important steps is choosing the appropriate libraries and frameworks that support cross-platform development in C. These tools can help developers avoid platform-specific issues, while still delivering applications that meet the stringent performance requirements of the crypto world. Below are some key considerations and recommendations for building efficient cross-platform applications in C for the cryptocurrency industry.
Essential Tools for Cross-Platform C Development
- Cross-Platform Libraries: Libraries such as libcurl and OpenSSL are vital for handling network communications and encryption, which are essential in cryptocurrency applications.
- Build Systems: Tools like CMake help automate the building process for multiple platforms, ensuring that the application compiles and runs correctly on all systems.
- Cross-Platform Compilers: Using compilers like GCC and Clang enables developers to compile C code on different platforms without manual adjustments.
Best Practices for Crypto Application Development
- Platform-Independent Code: Always aim for writing modular and platform-independent code. This will reduce platform-specific adjustments and enhance maintainability.
- Security Measures: In crypto applications, security is paramount. Be sure to use secure coding practices, such as input validation, secure memory handling, and encryption protocols.
- Testing Across Platforms: Regularly test your application on different operating systems to catch potential issues early and ensure compatibility.
Tip: Keep your codebase clean and use version control (e.g., Git) to track changes across platforms. This makes managing different versions of the application easier and more efficient.
Common Challenges in Cross-Platform Crypto Development
Challenge | Solution |
---|---|
Platform-Specific API Differences | Use conditional compilation and abstract the platform-specific APIs into separate modules. |
Performance Bottlenecks | Profile and optimize your code regularly, focusing on areas critical to performance such as cryptographic operations. |